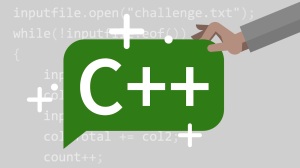
The only way to learn how to program is to write programs. You’ll learn a lot more by writing and debugging programs than you ever will by reading this book. This book contains many programming exercises, and you should try to do as many of them as possible. When doing the exercises keep good programming style in mind. Always comment your programs, even if you’re doing the exercises only for yourself. Commenting helps you organize your thoughts,
and commenting your own programs is good practice for when you go into the “real world.” Don’t let yourself be seduced by the idea that, “I’m only writing these programs for myself, so I don’t need to comment them.” First of all, code that looks obvious to you when you write it can often be confusing and cryptic when you revisit it a week later. Writing comments also helps you organize your ideas. (If you can write out an idea in English, you are halfway to writing it in C++.) Finally, programs tend to be around far longer than expected. I once wrote a program that was designed to work only on the computer at Caltech. The program was highly system dependent. As I was the only one who would ever use the program, the program would print the following message if I got the command line wrong
The Basics of Program Writing
Computers are very powerful tools that can store, organize, and process a tremendous amount of information. However, they can’t do anything until someone gives them detailed instructions.
Communicating with computers is not easy. They require instructions that are exact and detailed. Wouldn’t life be easier if we could write programs in English? Then we could tell the
computer, “Add up all my checks and deposits, and then tell me the total,” and the machine would balance our checkbooks.
But English is a lousy language when you must write exact instructions. The language is full of ambiguity and imprecision. Grace Hopper, the grand old lady of computing, once commented
on the instructions she found on a bottle of shampoo:
Wash
Rinse
Repeat
She tried to follow the directions, but she ran out of shampoo. (Wash-rinse-repeat. Wash-rinse-repeat. Wash-rinse-repeat. . . .)
Of course, we can try to write in precise English. We’d have to be careful and make sure to spell everything out and be sure to include instructions for every contingency. If we worked really hard, we could write precise English instructions, right?
As it turns out, there is a group of people who spend their time trying to write precise English. They’re called the government, and the documents they write are called government regulations.
Unfortunately, in their effort to make the regulations precise, the government also has made the documents almost unreadable. If you’ve ever read the instruction book that comes with your tax
forms, you know what precise English can be like.
Still, even with all the extra verbiage the government puts in, problems can occur. A few years ago California passed a law requiring all motorcycle riders to wear a helmet. Shortly after this
law went into effect a cop stopped a guy for not wearing a helmet. The man suggested the police officer take a closer look at the law.
The law had two requirements: 1) that motorcycle riders have an approved crash helmet and
2) that it be firmly strapped on. The cop couldn’t give the motorcyclist a ticket because the man did have a helmet firmly strapped on—to his knee.
So English, with all its problems, is out as a computer language. Now, how do we communicate with a computer?
The first computers cost millions of dollars, while at the same time a good programmer cost
about $15,000 a year. Programmers were forced to program in a language where all the instructions were reduced to a series of numbers, called machine language. This language could be directly input into the computer. A typical machine-language program looks like:
1010 1111
0011 0111
0111 0110
.. and so on for several hundred instructions
Whereas machines “think” in numbers, people don’t. To program these ancient machines, software engineers would write out their programs using a simple language where each word would stand for a single instruction. This was called assembly language because the programmers had to manually translate, or assemble, each line into machine code.
A typical program might look like:
Program Translation
MOV A,47 1010 1111
ADD A,B 0011 0111
HALT
0111 0110
.. and so on for several hundred instructions
Translation was a difficult, tedious, exacting task. One software engineer decided this was a perfect job for a computer, so he wrote a program, called an assembler, that would do the Job automatically.
He showed his new creation to his boss and was immediately chewed out: “How dare you even think of using such an expensive machine for a mere ‘clerical’ task?” Given the cost of an hour of computer time versus the cost of an hour of programmer’s time, this was not an unreasonable attitude. Fortunately, as time passed the cost of programmers went up and the cost of computers went
down. So it became more cost-effective to let the programmers write programs in assembly language and then use a program called an assembler to translate the programs into machine language.
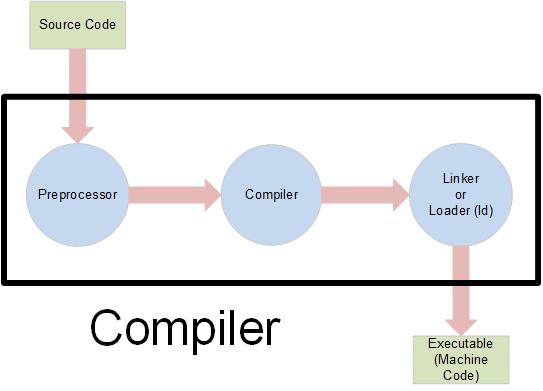
Programs from Conception to Execution
C++ programs are written in a high-level language using letters, numbers, and the other symbols you find on a computer keyboard. Computers actually execute a very low-level language called machine code (a series of numbers). So, before a program can be used, it must undergo several transformations. Programs start out as an idea in a programmer’s head. He writes down his thoughts in a file, called a sourcefile or source code, using a text editor. This file is transformed by the compiler into an objectfile. Next a program called the linker takes the object file, combines it with predefined routines from a standard library, and produces an executable program (a set of machine-language instructions). In the following sections, you’ll see how these various forms of the program work together to produce the final program.
Wrappers
Fortunately you don’t have to run the compiler, assembler, and linker individually. Most C++ compilers use “wrapper” programs, which determine which tools need to be run and then run them.
Some programming systems go even farther and provide the developer with an integrated development environment (IDE). The IDE contains an editor, compiler, linker, project manager, debugger, and more in one convenient package. Both Borland and Microsoft provide IDES with their compilers.
Creating a Real Program
Before you can actually start creating your own programs you need to know how to use the basic programming tools. This section will take you step by step through the process of entering, compiling, and running a simple program.
This section describes how to use two different types of compilers. The first type is the standalone or command-line compiler. This type of compiler is operated in a batch mode from the command line. In other words, you type a command and the compiler turns your source code into an executable program. The other type of compiler is contained in an IDE.
Most UNIX systems use command-line compilers. A few IDE-type compilers are available for UNIX, but they are rare. On the other hand almost all the compilers used with MS-DOS and Windows contain an integrated development environment. For command-line die-hards, these compilers do contain command-line compilers as well.
Hello World In C++
#include <iostream.h>
int main()
{
cout << “Hello World\n”;
return (0);
}